Coding
Project program code
Arduino cc software is used to write a program.
// The libraries used
#include "Adafruit_MQTT.h"
#include "Adafruit_MQTT_Client.h"
#include <WiFi.h>
// Define variables
int ThermistorPin = A0;
int Vo;
float R1 = 10000;
float logR2, R2, T;
float c1 = 0.001129148, c2 = 0.000234125, c3 = 0.0000000876741; // Steinhart-hart thermistor coefficients
// Defining connection parameters with Adafruit IO
#define AIO_SERVER "io.adafruit.com"
#define AIO_SERVERPORT 1883
#define AIO_USERNAME "vaneska98"
#define AIO_KEY "aio_EOgl90jflWRIZQzE7HShKeeswYCX"
WiFiClient client;
// Connection block with Adafruit IO
Adafruit_MQTT_Client mqtt(&client, AIO_SERVER, AIO_SERVERPORT, AIO_USERNAME, AIO_KEY);
boolean MQTT_connect();
boolean MQTT_connect() {
int8_t ret;
if (mqtt.connected()) {
return true;
} uint8_t retries = 3;
while ((ret = mqtt.connect()) != 0) {
mqtt.disconnect();
delay(2000);
retries--;
if (retries == 0) {
return false;
}
} return true;
}
// Adafruit IO connection function and an instruction to which account and board to send the data
Adafruit_MQTT_Publish tempsensor = Adafruit_MQTT_Publish(&mqtt, AIO_USERNAME
"vaneska98/feeds/tempsensor");
// Sensor control unit
void setup()
{
Serial.begin(115200);
WiFi.begin("Dinko", "12345678"); // Network name and password
while ((!(WiFi.status() == WL_CONNECTED))) { // We cycle here until we connect
delay(500);
Serial.print("Connection...");
}
// Print to the serial monitor just for information on what is happening
Serial.println("A connection has been established.");
Serial.println("Your IP is:");
Serial.println((WiFi.localIP()));
}
void loop()
{
Vo = analogRead(ThermistorPin);
R2 = R1 * (1023.0 / (float)Vo - 1.0); // We calculate the resistance of the thermistor
logR2 = log(R2);
T = (1.0 / (c1 + c2 * logR2 + c3 * logR2 * logR2 * logR2)); // The temperature in Kelvin
T = T - 273.15; // Conversion from Kelvin to Celsius
if (MQTT_connect()) { // We are checking if the connection to Adafruit IO is OK
if (tempsensor.publish(T)) { // If e OK we send the value of T
Serial.print("Temperature: ");
Serial.print(T);
Serial.println(" C");
} else { // Connection to Adafruit IO failed
Serial.println("There is a problem with the sensor or with the driver Adafruit");
}
}
delay(500);
}
How the program works (explanation from instructor is need)
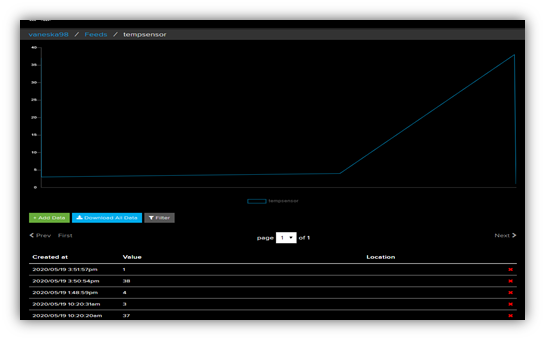
The resistance of the thermistor varies depending on the ambient temperature, we will use the Steinhart-Hart equation to derive the exact temperature of the thermistor. The code will return the temperature to Celsius.
Job test
In the dashboard, the tempsensor reports the value obtained from the code. After a few contacts, the schedule of events will begin to be built. Each event is documented with value, date, time and second.
E-mails received to report both events.
Conclusions
The following problems were considered:
- The communication capabilities of Arduino ESP32 modules;
- How to connect IoT to a database;
- How to create an IoT that sends data to a cloud database of Adafruit IO;
- Code measurements were created;
- What can we do with information in the cloud structure,
- Creating a trigger in IFTTT;
- A job test has been done.