Object Manipulation
Object Manipulation
The HelloAR sample let us place objects in scene. This sample, on the other hand, let's us not only add objects in scene, but also manipulate them such as:
- Manipulating objects to select them
- Moving objects around the scene
- Rotating objects
- Changing the size of an object
- Elevating an object
Now let's see what components the app uses.
- In the Unity Project window, navigate to Assets > GoogleARCore > Examples > ObjectManipulation > Scenes > ObjectManipulation.
- Double-click the ObjectManipulation scene to open it.
- Use the Hierarchy window to start navigating the sample scene.
Understanding the Code
Let's select Pawn Generator game object from Hierarchy. From Inspector, open the PawnManipulator script. Inside this script, we can decide if a specific gesture can be started, what to do while it progresses, and when it ends. In order to do this, we need to implement OnStartManipulation()
, OnContinueManipulation()
, and OnEndManipulation()
methods. We also must implement CanStartManipulationForGesture(TapGesture gesture)
method in order to start the manipulation when the user taps over an area that does not contain other objects.
The method CanStartManipulationForGesture(TapGesture gesture)
looks like this:
protected override bool CanStartManipulationForGesture(TapGesture gesture)
{
if (gesture.TargetObject == null)
{
return true;
}
return false;
}
null
.The
OnStartManipulation()
, OnContinueManipulation()
, and OnEndManipulation()
methods can look like this:protected override void OnEndManipulation(TapGesture gesture)
{
if (gesture.WasCancelled)
{
return;
}
// If gesture is targeting an existing object, we are done.
if (gesture.TargetObject != null)
{
return;
}
// Raycast against the location the player touched to search for planes.
TrackableHit hit;
TrackableHitFlags raycastFilter = TrackableHitFlags.PlaneWithinPolygon;
if (Frame.Raycast(gesture.StartPosition.x, gesture.StartPosition.y, raycastFilter, out hit))
{
// Use hit pose and camera pose to check if hit test is from the
// back of the plane, if it is, no need to create the anchor.
if ((hit.Trackable is DetectedPlane) &&
Vector3.Dot(FirstPersonCamera.transform.position - hit.Pose.position,
hit.Pose.rotation * Vector3.up) < 0)
{
Debug.Log("Hit at back of the current DetectedPlane");
}
else
{
// Instantiate game object at the hit pose.
var gameObject = Instantiate(PawnPrefab, hit.Pose.position, hit.Pose.rotation);
// Instantiate manipulator.
var manipulator =
Instantiate(ManipulatorPrefab, hit.Pose.position, hit.Pose.rotation);
// Make game object a child of the manipulator.
gameObject.transform.parent = manipulator.transform;
// Create an anchor to allow ARCore to track the hitpoint as understanding of
// the physical world evolves.
var anchor = hit.Trackable.CreateAnchor(hit.Pose);
// Make manipulator a child of the anchor.
manipulator.transform.parent = anchor.transform;
// Select the placed object.
manipulator.GetComponent<Manipulator>().Select();
}
}
}
ARCorePAwn
is instantiated as the child of a ManipulatorPrefab
. This prefab contains several Manipulator
scripts which extend the base Manipulator
class, overriding the callback functions mentioned in the previous bullet for different gesture types to implement the different behaviors to which the pawn is expected to react:
- SelectionManipulator
- TranslationManipulator
- ScaleManipulator
- RotationManipulator
- ElevationManipulator
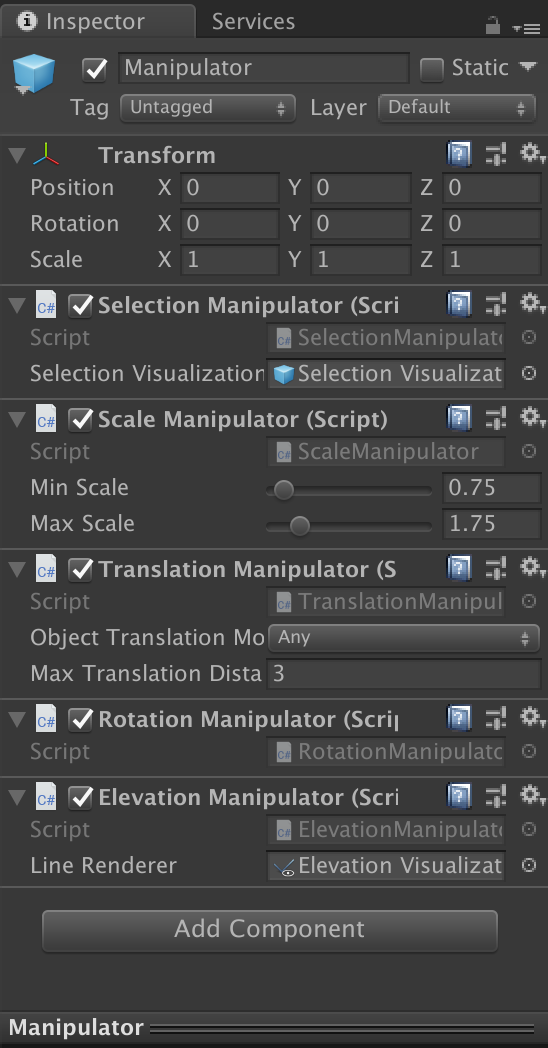
Manipulator
prefab can be selected at a given time, and it will be affected by the user's gestures to scale, rotate, or elevate it.