Seven-segment display
Create new circuit and call it "Seven-segment display".
Add components:
- Arduino Uno R3 board
- Resistor (1 kohms)
- Seven-segment display
- Breadboard
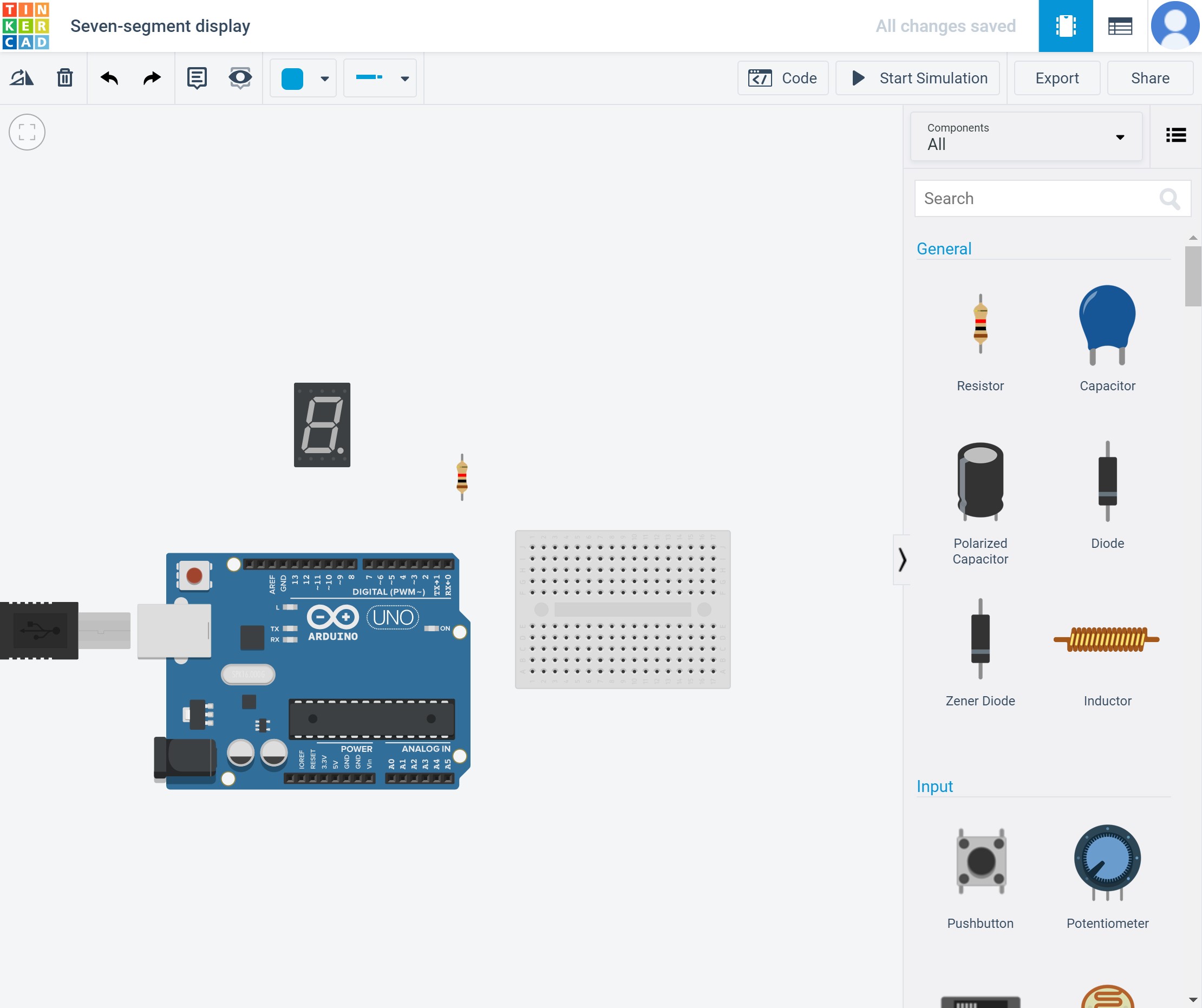
Determine if a display is common anode or common cathode
You can probe the pins with a test circuit constructed like this:
Connect the ground (black) wire to any pin of the display. Then insert the positive (red) wire into each one of the other pins. If no segments light up, move the ground wire over to another pin and repeat the process. Do this until at least one segment lights up.
When the first segment lights up, leave the ground wire where it is, and connect the positive wire to each one of the other pins again. If a different segment lights up with each different pin, you have a common cathode display. The pin that’s connected to the ground wire is one of the common pins. There should be two of these.
If two different pins light up the same segment, you have a common anode display. The pin that’s connected to the positive wire is one of the common pins. Now if you connect the ground wire to each one of the other pins, you should see that a different segment lights up with each different pin.
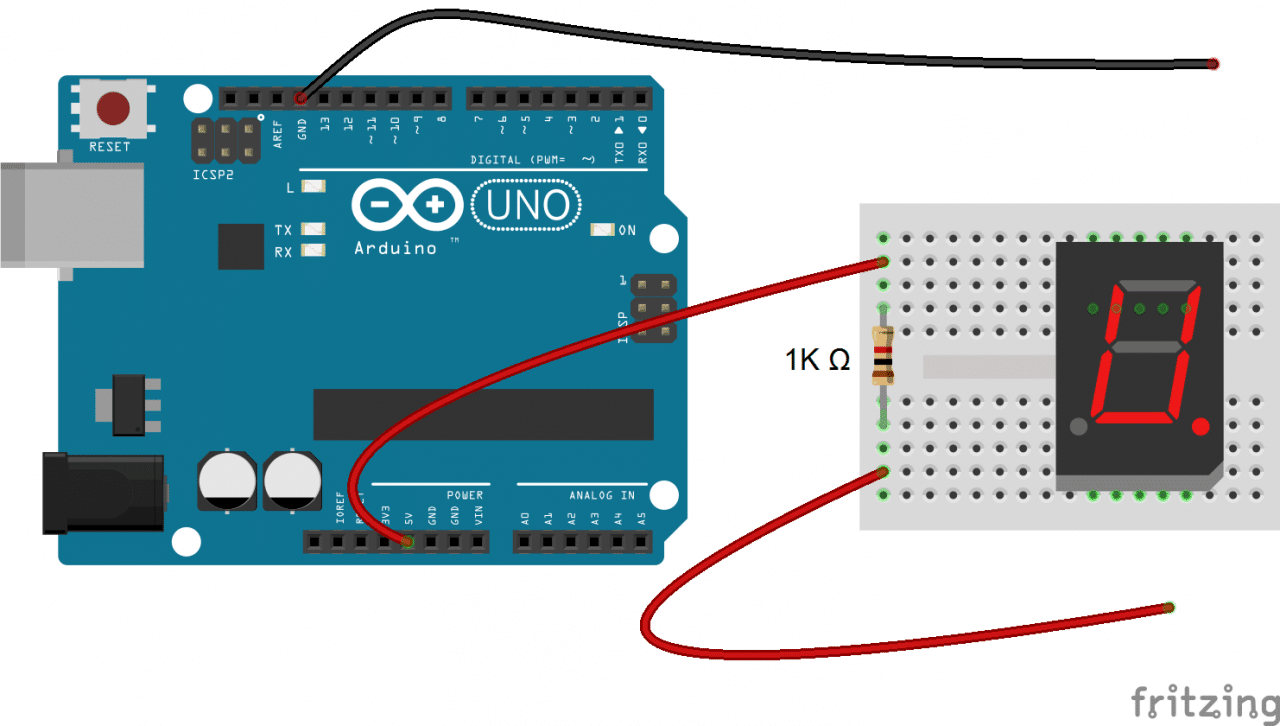
In this example we have common anode display. How to check it, watch in gif below.
Determine the pinout for your display
Now draw a diagram showing the pins on your display. With the common pin connected to the ground wire (common cathode) or positive wire (common anode), probe each pin with the other wire. When a segment lights up, write down the segment name (A-G, or DP) next to the corresponding pin on your diagram.
Connect components:
- Place seven-segment display and resistor on breadbord;
- Connect both common anode to resistor;
- Connect resistor to 5V;
- Connect each segment to Arduino Uno R3 digital pins as shown in the pinout diagram.
Code:
First, we need to define variables. The output corresponding to each segment will be called the same as the segment.
int a = 8; //For displaying segment "a"
int b = 9; //For displaying segment "b"
int c = 3; //For displaying segment "c"
int d = 4; //For displaying segment "d"
int e = 5; //For displaying segment "e"
int f = 7; //For displaying segment "f"
int g = 6; //For displaying segment "g"
int dp = 2; //For displaying dot
Now we can go to the function setup () and define variables as output.
void setup() {
pinMode(a, OUTPUT); //A
pinMode(b, OUTPUT); //B
pinMode(c, OUTPUT); //C
pinMode(d, OUTPUT); //D
pinMode(e, OUTPUT); //E
pinMode(f, OUTPUT); //F
pinMode(g, OUTPUT); //G
pinMode(dp, OUTPUT); //Dot
}
In function loop () write your code and try to start the simulation.For example:
void loop() {
digitalWrite(a, HIGH);
}
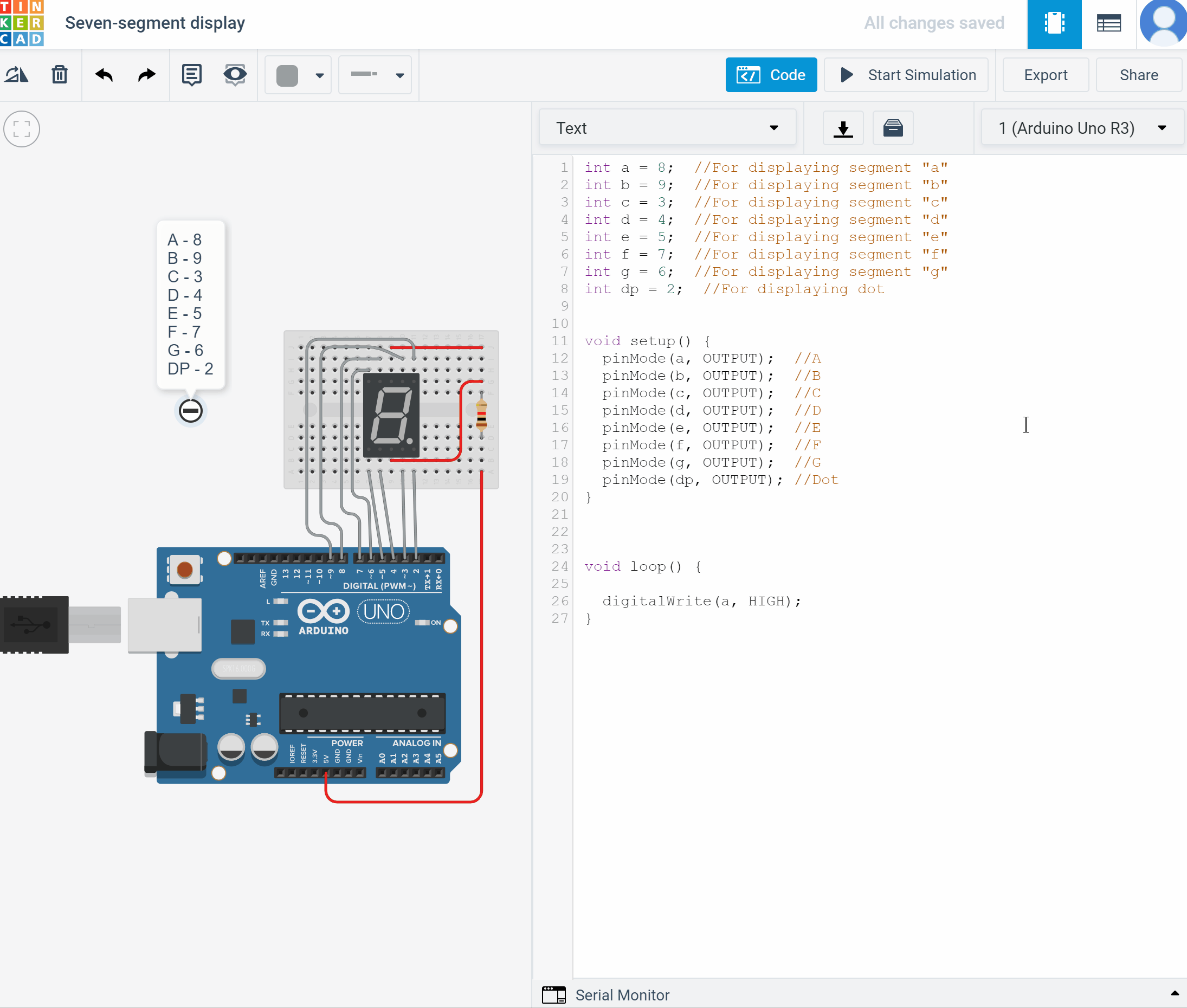
You can see in the simulation that the only segment that doesn't work is A.
Why?
We have common anode display. When our pins are on the low state, segments are working. Let's change the code.
void loop() {
digitalWrite(a, LOW);
digitalWrite(b, HIGH);
digitalWrite(c, HIGH);
digitalWrite(d, HIGH);
digitalWrite(e, HIGH);
digitalWrite(f, HIGH);
digitalWrite(g, HIGH);
digitalWrite(dp, HIGH);
}
Now you see, that only segment that is working are A.
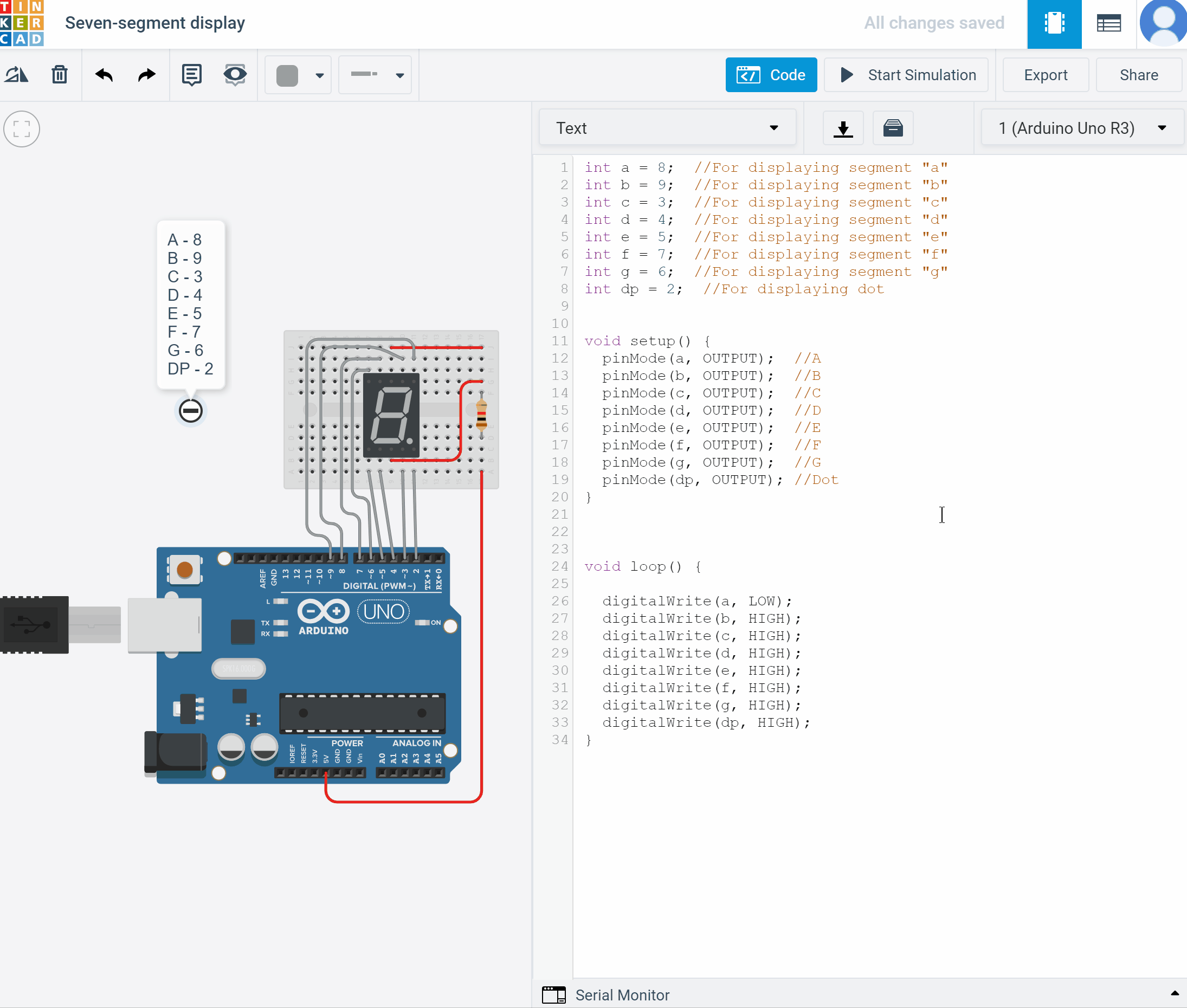
Let's create a function for turning off all segments.
Write this function before function setup().
void turnOff() {
digitalWrite(a,HIGH);
digitalWrite(b,HIGH);
digitalWrite(c,HIGH);
digitalWrite(d,HIGH);
digitalWrite(e,HIGH);
digitalWrite(f,HIGH);
digitalWrite(g,HIGH);
digitalWrite(dp,HIGH);
}
Add our new function to function loop().
void loop() {turnOff();
digitalWrite(a, LOW);
}
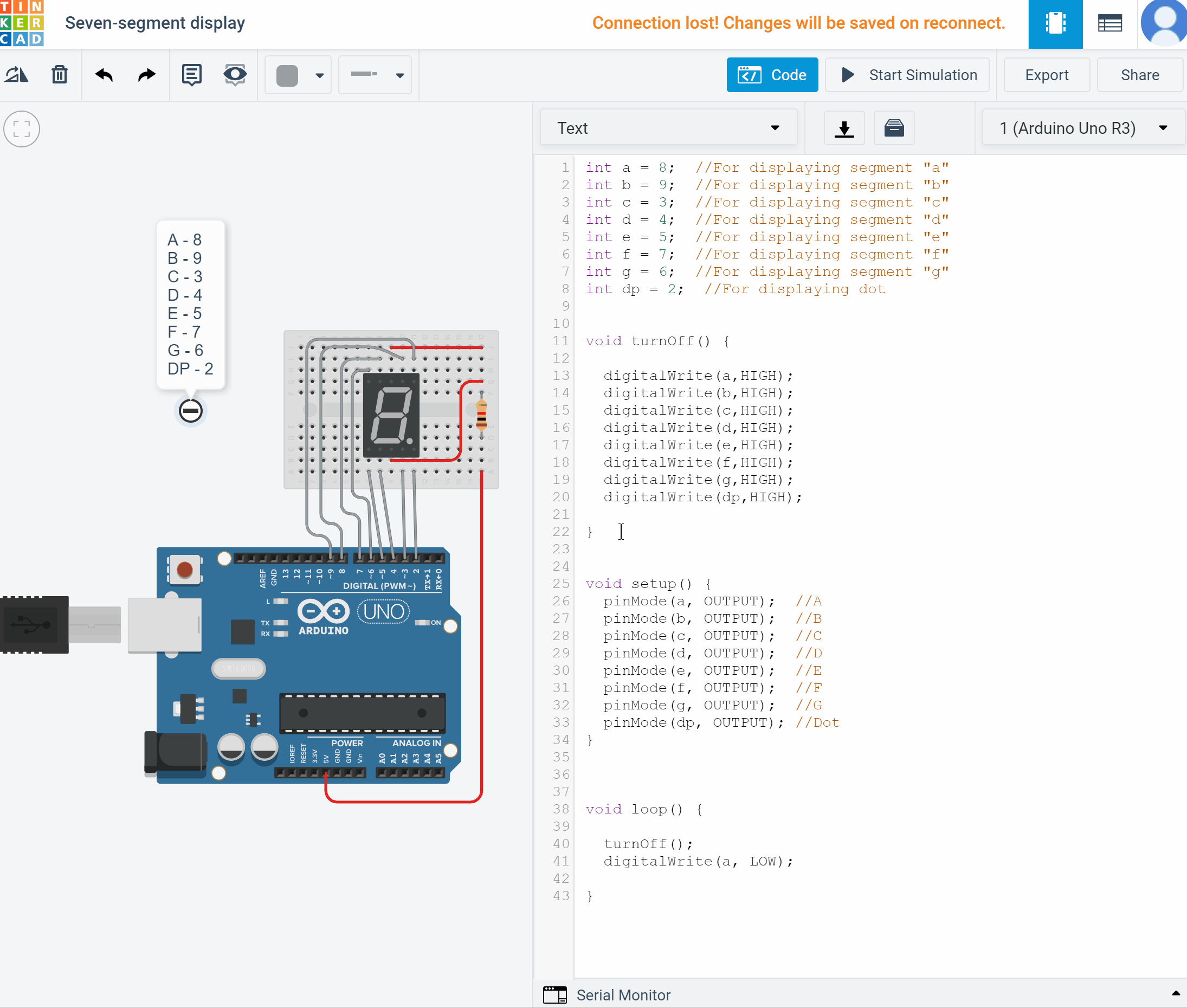
Now you can turn on any sections and make numbers on your own!
Feel free to add your own code!
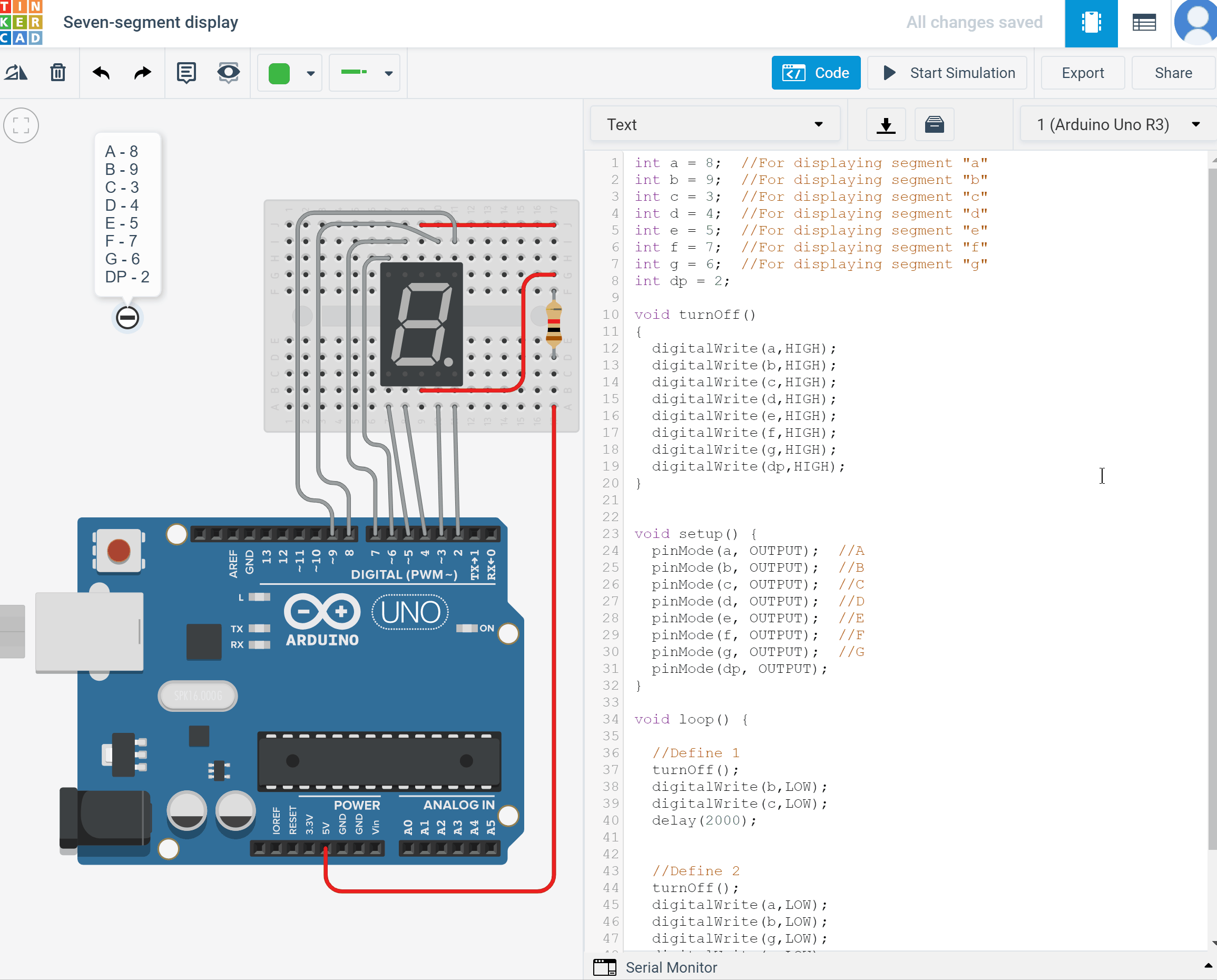