First Person/Third Person Camera
First Person Camera. Third Person Camera
In many games we have seen that we can either control a character (the camera follows the character - third person camera) or we are the character (the camera sees through the character's eyes - first person camera). Let's see how we can implement this.
In order to do this, we will need to have 2 cameras in scene, only one active at a time. One camera will be positioned as a first person camera and the other as a third person camera.
1. Rename Main Camera to First Person Camera.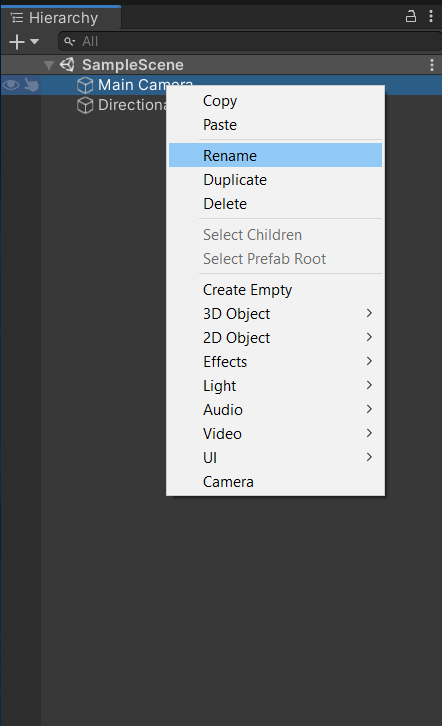
2. Create a new camera and name it Third Person Camera.
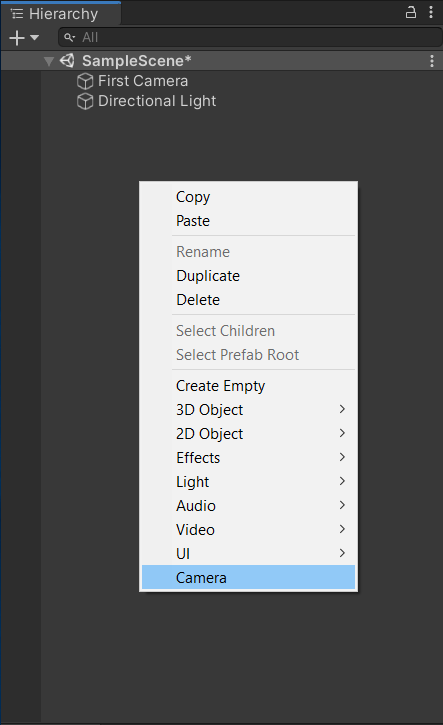
3. Create a new script.
4. Inside the script declare the two cameras.
5. In the Start() method, set which camera is active and which is not active.
6. In Update(), check which camera is active and update it's position depending on the position of the player. For the first person camera the position will be the same as the character's position. For the third person camera, the position will be in the back of the player, so we will add something to the character's position.
7. We want to switch between the cameras when we press space, so in Update() we will also check this.
8. Attach the script to the character's game object.
9. Map the 2 cameras from the script to the two cameras from hierarchy. Drag and drop each camera.
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class CameraSwitch: MonoBehaviour
{
public Camera firstPersonCam;
public Camera thirdPersonCam;
private float rotX = 0.0f;
private float rotY = 0.0f;
public float mouseSensitivity = 100.0f;
public float clampAngle = 80.0f;
Vector3 offset;
// Start is called before the first frame update
void Start()
{
thirdPersonCam.enabled = true;
firstPersonCam.enabled = false;
offset = new Vector3(0.0f, 0.0f, -2.0f);
rotX = transform.localRotation.eulerAngles.x;
rotY = transform.localRotation.eulerAngles.y;
}
// Update is called once per frame
void Update()
{
float mouseX = Input.GetAxis("Mouse X");
float mouseY = -Input.GetAxis("Mouse Y");
rotY += mouseX * mouseSensitivity * Time.deltaTime;
rotX += mouseY * mouseSensitivity * Time.deltaTime;
rotX = Mathf.Clamp(rotX, -clampAngle, clampAngle);
Quaternion localRotation = Quaternion.Euler(rotX, rotY, 0.0f);
transform.rotation = localRotation;
if (thirdPersonCam.enabled == true && firstPersonCam.enabled == false)
{
thirdPersonCam.gameObject.transform.position = transform.position + localRotation * offset;
thirdPersonCam.gameObject.transform.LookAt(transform.position);
}
if (thirdPersonCam.enabled == false && firstPersonCam.enabled == true)
{
firstPersonCam.gameObject.transform.eulerAngles = transform.eulerAngles;
firstPersonCam.gameObject.transform.position = transform.position;
}
if (Input.GetKeyDown(KeyCode.Space))
{
if (thirdPersonCam.enabled == true)
{
thirdPersonCam.enabled = false;
firstPersonCam.enabled = true;
}
else
{
thirdPersonCam.enabled = true;
firstPersonCam.enabled = false;
}
}
}
}
Ultima modificare: Saturday, 24 July 2021, 17:16