Coding. How to send data to the cloud
Project
program code
The Arduino.cc software was used to write the program.
////////////////////////////////////
// IoT_BFU //
// Access sensor sends data //
// In Adafruit IO Cloud Database //
////////////////////////////////////
// Enable Adafruit IO libraries and network connectivity
#include "Adafruit_MQTT.h"
#include "Adafruit_MQTT_Client.h"
#include <WiFi.h>
int i; // Variable in which we will store the values from the sensor
// Define Adafruit IO connection parameters
#define AIO_SERVER "io.adafruit.com"
#define AIO_SERVERPORT 1883
#define AIO_USERNAME "IoT_BFU"
#define AIO_KEY "aio_vbEQ73TLu64IPZXfgIMzxxxxxxxxxx"
WiFiClient client;
// Adafruit IO connectivity block
Adafruit_MQTT_Client mqtt(&client, AIO_SERVER, AIO_SERVERPORT, AIO_USERNAME, AIO_KEY);
boolean MQTT_connect();
boolean MQTT_connect() {
int8_t ret;
if (mqtt.connected()) {
return true;
} uint8_t retries = 3;
while ((ret = mqtt.connect()) != 0) {
mqtt.disconnect();
delay(2000);
retries--;
if (retries == 0) {
return false;
}
} return true;
}
// Adafruit IO contact feature and indication to which account and dashboard to send the data
Adafruit_MQTT_Publish touchsensor = Adafruit_MQTT_Publish(&mqtt, AIO_USERNAME "/feeds/touchsensor");
// Sensor and LED control unit
void setup()
{
i = 0;
Serial.begin(115200);
pinMode(2, OUTPUT);
digitalWrite (2, HIGH); // Control the LED
delay(300);
digitalWrite(2, LOW);
delay(3000);
Serial.println("START");
WiFi.begin (" Valentina ", " xxxxxxxxxxxxxx "); // Network name and password
while ((! ( WiFi.status () == WL_CONNECTED))) { // loop here until we connect
delay(500);
Serial.print ("Connecting ...");
} // Print to serial monitor just for info on what's going on
Serial.println ("Connected.");
Serial.println ("Your IP is:");
Serial.println((WiFi.localIP()));
Serial.println ("Sensor touch is checked");
}
void loop()
{
i = ( touchRead (T0)); // We assign the value read from the sensor to i
if (( touchRead (T0)) <20) { // We check that it is less than 20
if ( MQTT_connect ()) { // Checking if Adafruit IO connection is OK
if ( touchsensor.publish (i)) { // If e OK we send the value of i
Serial.print ("CONTACT WITH SENSOR IS REPORTED. CONTACT LEVEL:");
Serial.println(i);
digitalWrite(2, HIGH);
delay(1000);
digitalWrite(2, LOW);
} else { // Adafruit IO connection failed
Serial.println ("There is a problem with the sensor or the Adafruit driver");
}
}
} else { // Value of i> 20 I only print to the serial monitor
Serial.print ("No contact. Reported contact level is:");
Serial.println(i);
}
delay (1000); // Delay - 1 second
}
////////////////////////////////////////////////////
Result of serial monitor performance
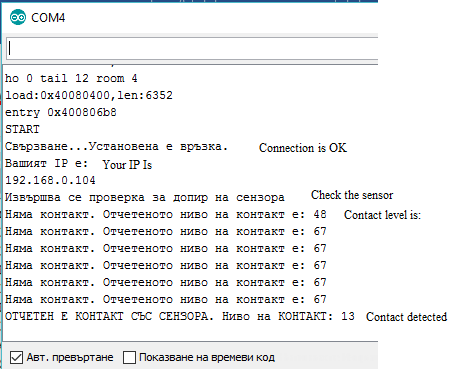
When you touch the touch sensor, a message will appear about the contact level counted from 0 to 100.
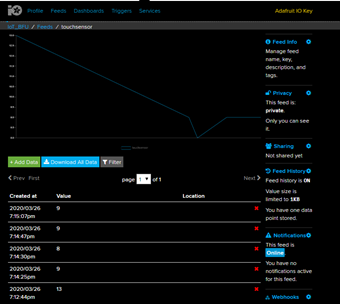