User Interface. Creating and binding new Scenes
Creating and binding new scenes
Games usually have multiple scenes: a main menu scene, a game over scene, a level 1 scene, a level 2 scene etc. Usually we create a new scene when we need to add completely new content on the scene. Let's see how we can do that.
1. Right click in the assets menu -> Create -> Scene.
2. Go to File -> Build Settings. Click on Add Open Scenes button. Make sure the current scene is checked. Close the window. Remember the index of the current scene.
3. Click on the newly created scene. If you haven't already done so, Unity will ask you to save the current scene.
4. Repeat Step 2 for the newly created scene. Don't forget the index for the new scene.
5. If we want to change between scenes (for instance, when a button is pressed), we need to add the following code:
SceneManager.LoadScene(1);
The number inside the brackets represents the index we had to remember at Step 2. However, in order to be able to use this function, we need to include a new library:
using UnityEngine.SceneManagement;
User Interface
The user interface represents in a computer game all the elements which are meant for the player and not for the character. For instance, a button, a text, a text input etc. Let's see how we can use such elements.Firstly, let's create a button in the main menu scene which will start the game.
1. In Hierarchy create a button: right click -> UI -> Button.
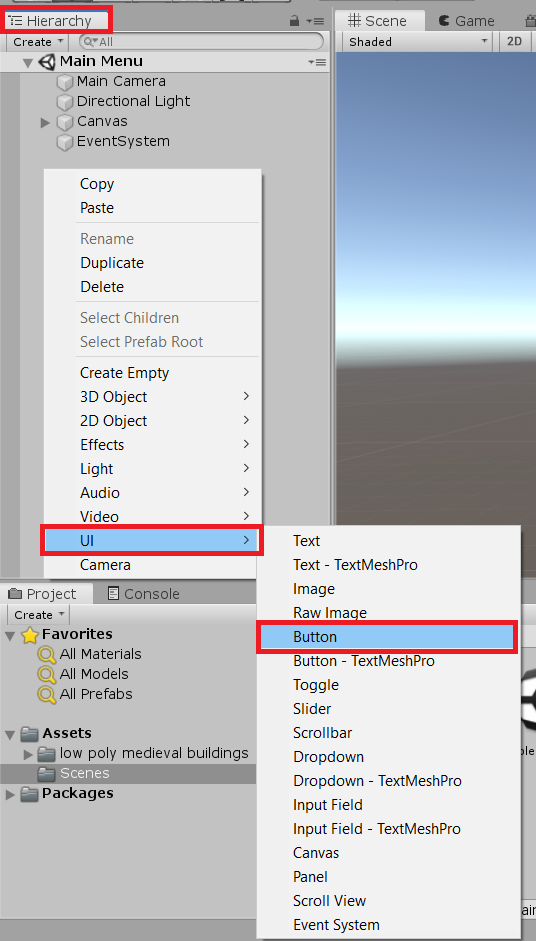
2. Probably you have noticed that besides the button, a Canvas element and an EventSystem object were also created. In order to be able to display user interface elements on screen, we need a canvas. All the elements placed on the canvas, will be displayed on screen. Th EventSystem object is used to register specific user interface events (such as a button was pressed).
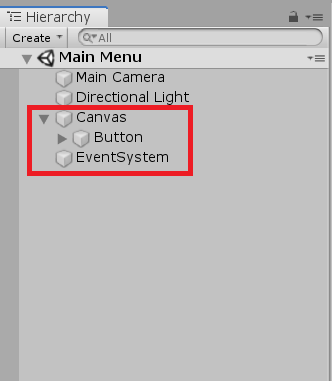
3. Create and add a new script on the EventSystem game object. Let's call the script ButtonManager.
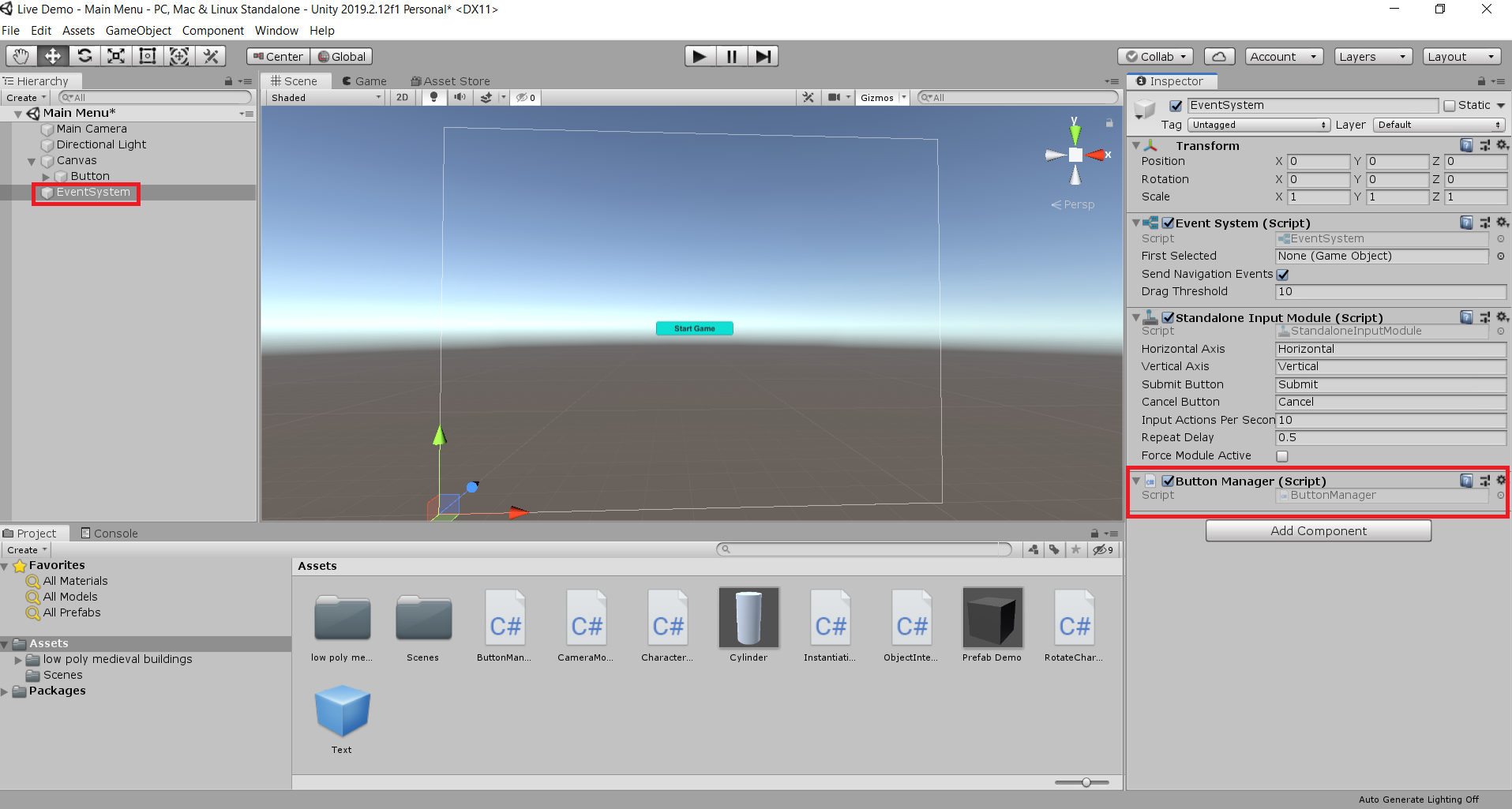
4. Inside the script, create a public function which will open the other scene. The function should be public so that we can see it from the Unity interface. All the public variables and functions can be accessed and updated directly from Unity. Let's call the function Loading.
5. The Button game object contains an On Click() list. Click on the plus sign. Drag and drop the EventSystem component in the third newly added cell. Then in the second cell look for ButtonManager script and then for the Loading method. Now we have mapped out button to our function.
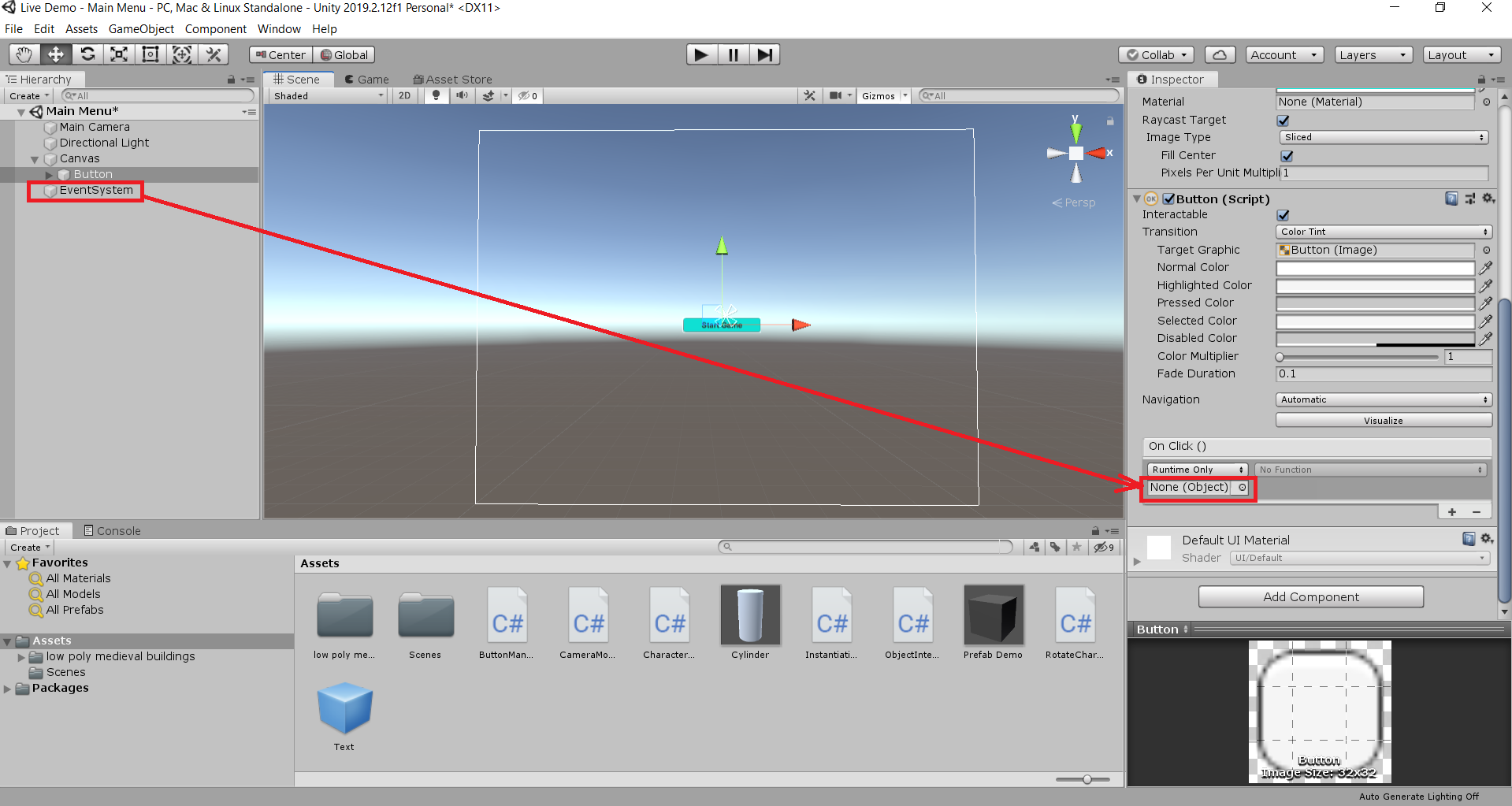
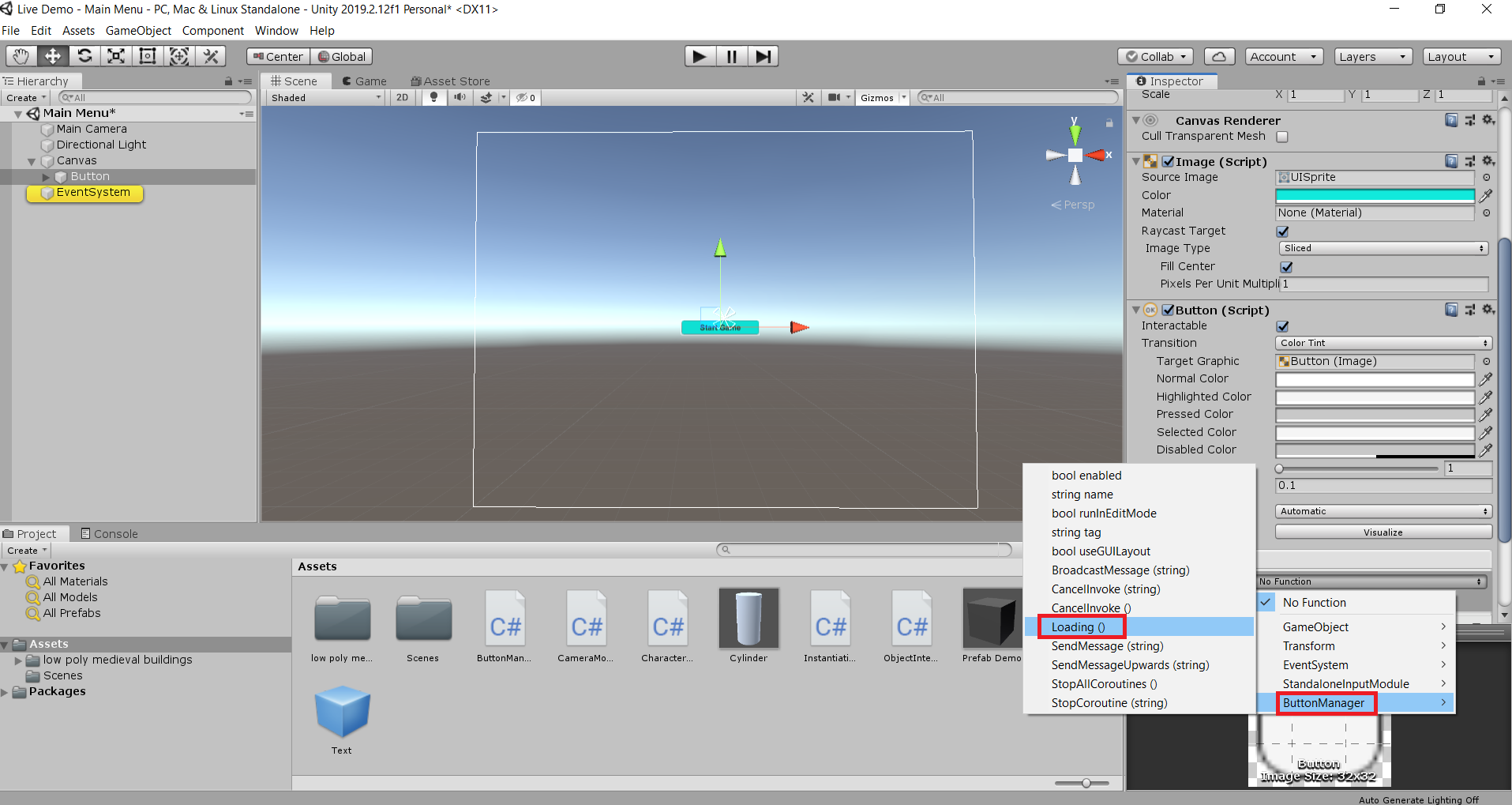
6. Let's change the message on the button. In Hierarchy, click on the text of the button. In Inspector change the text component of the button with the message you want.
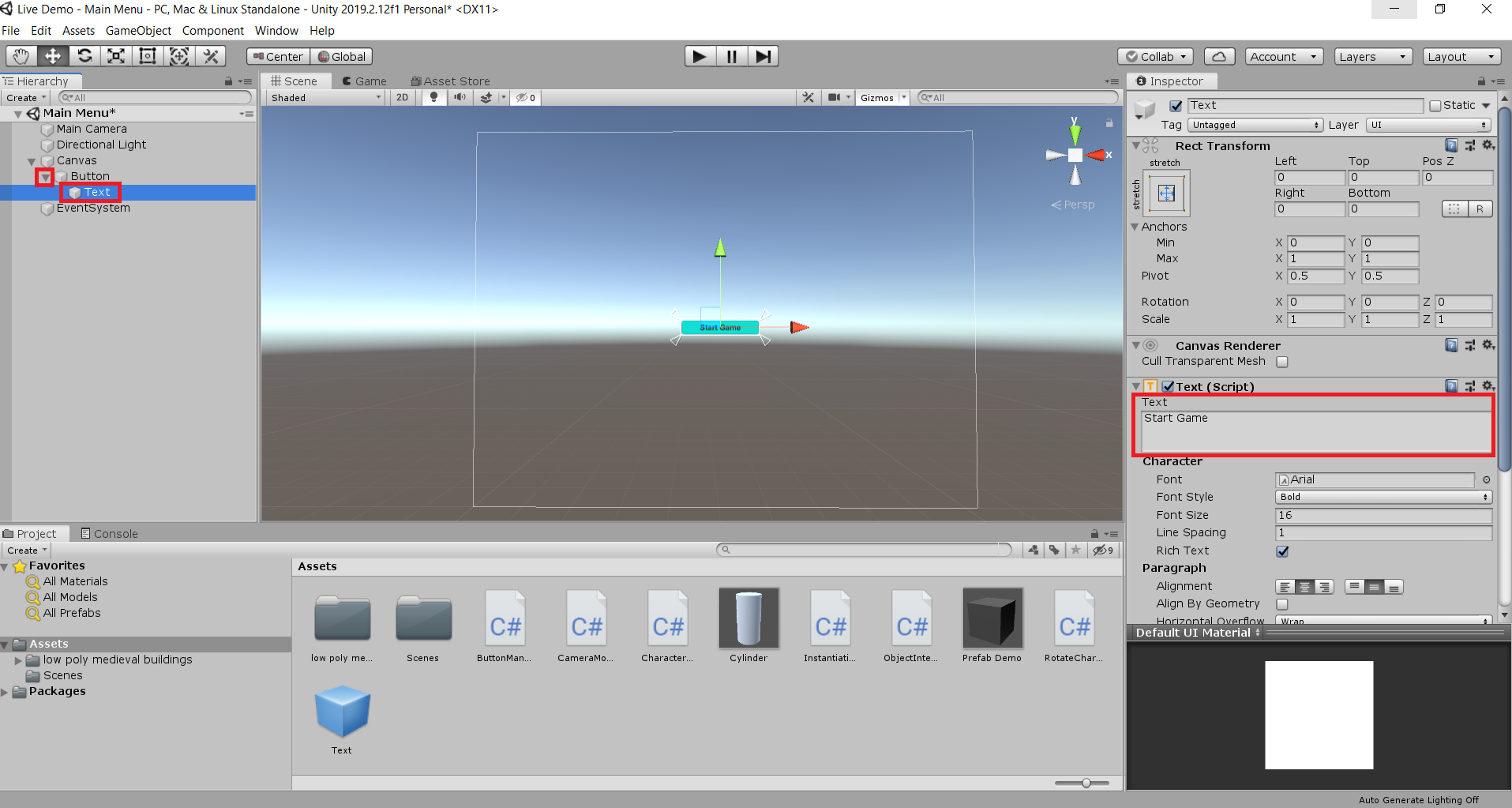
This is how the code should look like:
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.SceneManagement;
public class ButtonManager : MonoBehaviour
{
// Start is called before the first frame update
void Start()
{
}
// Update is called once per frame
void Update()
{
}
public void Loading()
{
SceneManager.LoadScene(1);
}
}
Now let's display the score on the screen. Let's say that each time the player collects a prefab, the score increases.
1. Let's go back to the other scene.
2. In Hierarchy, add a new text element. Again, a Canvas and an EventSystem will be created.
3. Change the text of the Text component with something more suggetive. For instance: "Score: ".
4. Add a new Text component in scene and place to the right of the first Text component. The message of this text should be 0 (the initial value of the score).
5. Make the text a prefab.
6. Open the ObjectInteraction script.
7. Create a public Text variable, which will be used to update the score on screen.
8. Double click on the collectible prefab.
9. Drag and drop the text prefab on the ObjectInteraction script from the collectible prefab.
This is how the final code should look like:
using System.Collections;
using System.Collections.Generic;
using System;
using UnityEngine;
using UnityEngine.UI;
public class ObjectInteraction : MonoBehaviour
{
int score = 0;
public Text scoreText;
void Update()
{
score = Int32.Parse(scoreText.text);
}
IEnumerator DestroyObject()
{
yield return new WaitForSeconds(2.0f);
Destroy(gameObject);
score = score + 1;
scoreText.text = score.ToString();
}
void OnTriggerEnter(Collider other)
{
StartCoroutine(DestroyObject());
}
}
For more details, watch this video: